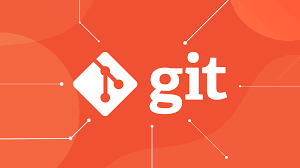
Some useful git tips:
Stash, Checkout, partially staging and MV
Stash
stash some files.
Used when you need pull changes from remote repository, but you made some changes locally that are not ready to be commited.
git stash # only unstaged
git stash -u # include untracked
recover files
git stash pop
Checkout
Change current branch:
git checkout branch_name
Clean all unstaged change and recover to last commit status:
git checkout .
Restore
Restore the staging area status of the file, but not change the file.
git restore --staged xxx
Restore a file to staging area status. If have not staged any changes, restore from HEAD (staging area status is same as HEAD).
git restore xxx
Reset
Withdraw a commit, retain changes
git reset --soft HEAD^
Delete a commit, delete all changes in this commit
git reset --hard HEAD
Revert
Patch staging
Stage part of a file.
Firstly,
git add -p file
Then press e to edit mode.
Then remove all changes you don’t want to make in this commit:
- Remove
-
cahges by replacing-
to ’ ’ (a space). - Remove
+
changes by commenting whold line.
MV
Move file xxx1 to xxx2. Both of the file and the git record will be removed.
git mv xxx1 xxx2
RM
Untrack a file:
git rm --cached xxx # this is a change, need commit it to make it effective
Untrack all .DS_Store:
find . -name .DS_Store -exec git rm --cached {} \;
Sub-module
Ad sub-module:
git submodule add <repo URL> <path>
# like:
git submodule add https://github.com/example/submodule.git externals/submodule
Remove sub-module:
# 1. Remove content in sub-module directory
git submodule deinit -f -- <path>
# 2. Edit .gitmodules, remove the sub-module in it
# 3. Edit .git/config, remove the sub-module in it
# 4. Stop tracking sub-module directory
git rm --cached <path>
# 5. Remove cached module and module folder
rm -rf .git/modules/<path>
rm -rf <path>
Clone repository with sub-module:
git submodule init
git submodule update
# Or
git clone --recurse-submodules
Hook
/.git/hooks/pre-push
hook example:
#!/bin/bash
# Path to the script that updates the last modification time
script_path="/path/to/update_lastmod.sh"
# Path to the folder you want to process
folder_path="/path/to/your/folder"
# Execute the script with the folder path as an argument
$script_path "$folder_path"
# If the script made changes, create a new commit
if ! git diff --quiet; then
git commit -am "Auto-commit: Update last modification times"
fi
# Continue with the push operation
exit 0
Then make the script executable:
chmod +x .git/hooks/pre-push
chmod +x /path/to/update_lastmod.sh