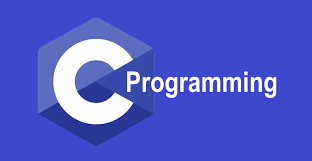
Cut out summary from your post content here.
C-style 2D array
-
Fully fixed
int static_arr[3][4]; for (i = 0; i < 3; i++) { for (j = 0; j < 4; j++) { printf("Address of static_arr[%d][%d]: %p\n", i, j, &static_arr[i][j]); } } printf("\n");
-
Fully free
int** fully_free_arr = (int **)malloc(3 * sizeof(int *)); for (i = 0; i < 3; i++) { dynamic_arr1[i] = (int *)malloc(4 * sizeof(int)); } for (i = 0; i < 3; i++) { for (j = 0; j < 4; j++) { fully_free_arr[i]+j = i*j; *(fully_free_arr+i)+j = i*j; //or fully_free_arr[i][j] = i*j; //or } } for (i = 0; i < 3; i++) { for (j = 0; j < 4; j++) { printf("Address of dynamic_arr1[%d][%d]: %p\n", i, j, &dynamic_arr1[i][j]); } }
-
Column fixed
-
Row fixed
NULL and nullprt
NULL
and nullptr
are both used in C++ programming to represent null pointer values, but there are key differences between them:
NULL
: This is a macro that is a null pointer constant. It’s a value that a pointer can take to represent that it’s not pointing to any valid memory location.NULL
is a carryover from C to C++ and is typically defined as0
or(void*)0
.nullptr
: This is a keyword introduced in C++11 to represent a null pointer.nullptr
is of typestd::nullptr_t
, which can be implicitly converted into any pointer type. It’s a special type that’s not an integer, and its purpose is to solve ambiguity problems that arise withNULL
.
Here’s an example that illustrates the problem that nullptr
solves:
void foo(int);
void foo(char*);
foo(0); // calls foo(int)
foo(NULL); // might call either foo depending on if NULL is defined as 0 or (void*)0
foo(nullptr); // unambiguously calls foo(char*)
In this case, nullptr
guarantees that the call will be made to foo(char*)
, whereas NULL
might not, depending on how it’s defined.